Part 3 - Enhancing Product Descriptions with OpenAI
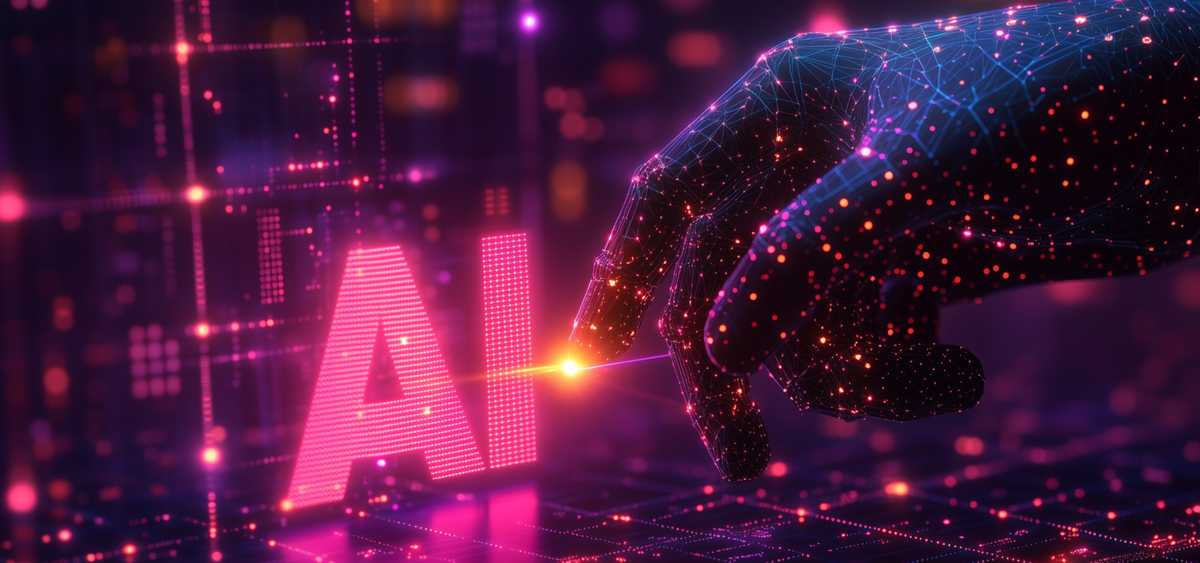
Welcome to part three of our journey into building a modern, intelligent RESTful API. So far, we’ve built a robust foundation with Node.js, Express, and PostgreSQL, creating a seamless marketplace of vendors and products. But what good is a marketplace if the product descriptions are bland and uninspiring? Enter OpenAI, the language model capable of transforming mundane product details into compelling, persuasive content that sells.
Imagine this: a simple product description—“Wooden Chair, sturdy and durable”—magically becomes, “Elegant Wooden Chair crafted for style and strength, designed to elevate your home with a touch of timeless beauty.” That’s the power of AI, and today, we’re integrating it into our API to give our products the spotlight they deserve.
Setting the Scene: Why Use OpenAI for Product Descriptions?
Writing great product descriptions is both an art and a science. They need to be engaging, informative, and persuasive, all while keeping the reader interested. But not every vendor has a copywriter on staff, and not every description can be perfect—until now.
By integrating OpenAI into our API, we can automatically rewrite and enhance product descriptions, turning simple phrases into marketing gold. And it all happens behind the scenes, with our Express API orchestrating the magic.
1400+ Free HTML Templates
334+ Free News Articles
63+ Free AI Prompts
299+ Free Code Libraries
51+ Free Code Snippets & Boilerplates for Node, Nuxt, Vue, and more!
25+ Free Open Source Icon Libraries
Step 1: Setting Up OpenAI
First, we need to install the openai
package, which will help us interact with OpenAI’s API:
npm install openai
Next, we’ll need an API key from OpenAI. Head over to OpenAI’s website to sign up and grab your API key. Once you have it, add it to your .env
file:
OPENAI_API_KEY=your_openai_api_key_here
Step 2: Configuring OpenAI in Your Project
Update your app.js
to include OpenAI’s configuration:
require('dotenv').config(); // Load environment variables
const express = require('express');
const { Pool } = require('pg');
const { Configuration, OpenAIApi } = require('openai');
const app = express();
const port = 3000;
// Set up the PostgreSQL connection
const pool = new Pool({
host: process.env.DB_HOST,
user: process.env.DB_USER,
password: process.env.DB_PASSWORD,
database: process.env.DB_NAME,
port: process.env.DB_PORT
});
// Set up OpenAI
const configuration = new Configuration({
apiKey: process.env.OPENAI_API_KEY,
});
const openai = new OpenAIApi(configuration);
app.use(express.json());
Now, we’re ready to use OpenAI to transform product descriptions.
Step 3: Writing the Function to Enhance Descriptions
Let’s create a route that updates a product’s description using OpenAI. When a product description is updated, we’ll call OpenAI’s API to rewrite it and then save the enhanced version back to the database.
app.put('/products/:id/description', async (req, res) => {
try {
const { id } = req.params;
const { description } = req.body;
// Call OpenAI to rewrite the description
const response = await openai.createCompletion({
model: 'text-davinci-003',
prompt: `Rewrite the following product description to make it more persuasive and attractive: "${description}"`,
max_tokens: 150,
});
const enhancedDescription = response.data.choices[0].text.trim();
// Update the product in the database
const result = await pool.query(
'UPDATE products SET description = $1 WHERE id = $2 RETURNING *',
[enhancedDescription, id]
);
res.json(result.rows[0]);
} catch (error) {
console.error('Error updating product description', error);
res.status(500).send('Internal Server Error');
}
});
Here’s what’s happening:
- Extracting the Product ID and Description: We use
req.params
andreq.body
to get the product ID and the original description. - Calling OpenAI: We make a request to OpenAI’s
text-davinci-003
model, asking it to rewrite the description. The prompt is simple but effective, andmax_tokens
controls the length of the output. - Saving the Enhanced Description: We update the
products
table with the rewritten description and return the updated product to the client.
Step 4: Testing the Magic
With your API running, test the /products/:id/description
route using a tool like Postman or cURL. Send a PUT
request with a sample product description, and watch as OpenAI transforms it into something compelling.
Example request:
{
"description": "A simple blue mug, perfect for everyday use."
}
And OpenAI might return:
“A beautifully crafted blue mug that adds a splash of color to your mornings, perfect for savoring your favorite coffee or tea every day.”
Now, that’s a description that makes you want to buy the mug!
The Benefits of Using OpenAI for Product Descriptions
- Consistency: Every product gets a polished, professional description, even if it started as a simple, unrefined phrase.
- Persuasiveness: OpenAI knows how to write copy that engages and converts, giving your products the attention they deserve.
- Efficiency: No need to spend hours crafting the perfect description. Your API does it for you, instantly.
For more tips on web development, check out DailySandbox and sign up for our free newsletter to stay ahead of the curve!
Comments ()