Mastering Data Sync in Postgres: Automating Resource Counts with Triggers
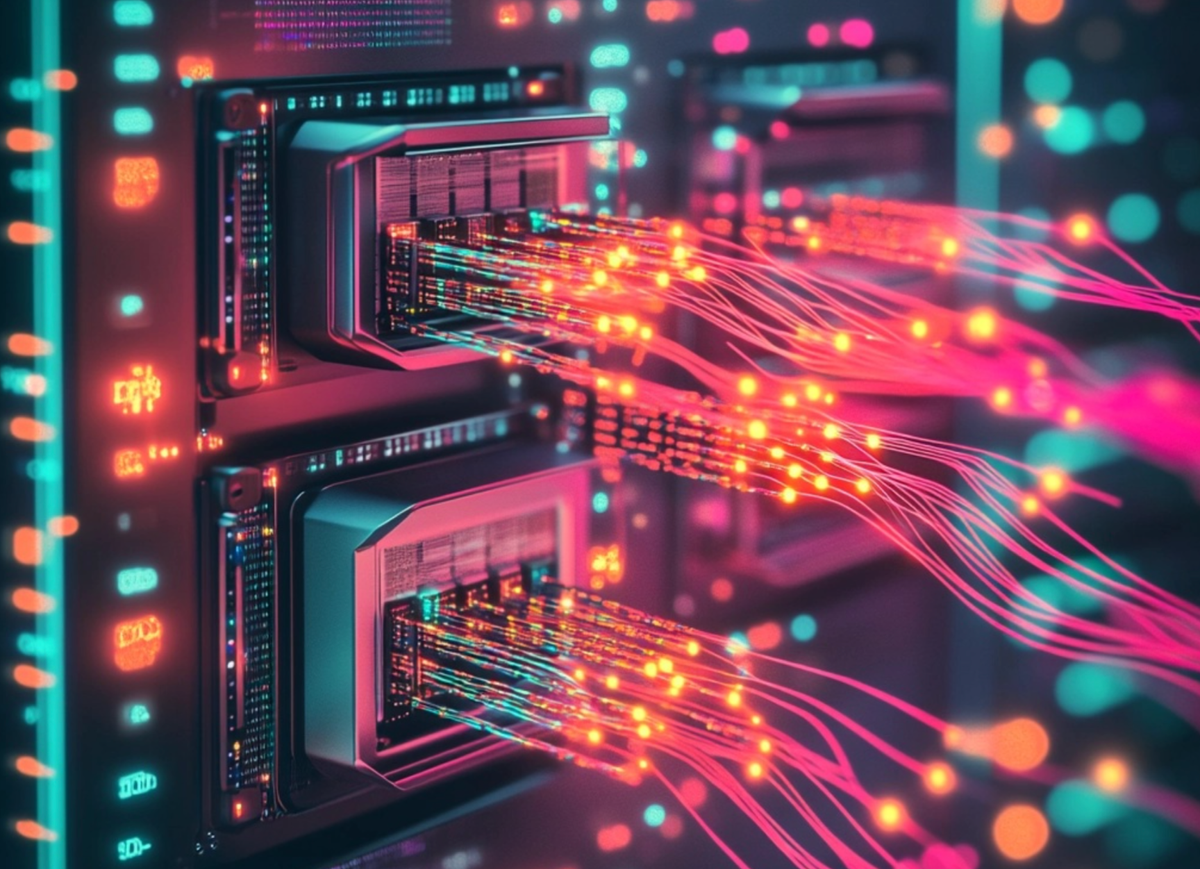
Imagine a library with thousands of shelves, each dedicated to a different type of resource—books, magazines, research papers, and more. Every time a new book is added, someone rushes to update the library’s main catalog, ensuring the count stays accurate. Now, translate that vision into the world of databases, where keeping track of your ever-growing collection of digital resources is just as critical. This is where Postgres triggers step in, like dutiful librarians, to keep everything in perfect sync.
The Heart of the System: The "Resources" Table
In our setup, there’s a master table called resources. It’s the ultimate catalog, holding key information about every category of content we manage: articles, boilerplates, templates, snippets, you name it. One crucial column in this table is total_num
, which records the total number of entries in each resource category.
The magic? We don’t manually update total_num
every time a new item is added. Instead, we let Postgres triggers do the heavy lifting, ensuring that the master catalog stays perfectly up-to-date with no room for human error.
The Grand Design: A Table for Every Resource
Our data structure is simple yet elegant. For each type of resource—be it articles, boilerplates, or templates—we have a corresponding table. Each of these tables holds entries specific to that resource type:
- articles: Stores all articles, complete with titles, content, and metadata.
- boilerplates: Houses reusable code snippets and projects.
- templates: Catalogs design templates, ready for use.
And the resources table? It’s our overseer, a one-stop reference for the total count of each resource type.
Enter Postgres Triggers: The Unseen Helpers
Postgres triggers are like invisible caretakers. Every time a new resource is added to one of the tables—say, a fresh article or a shiny new boilerplate—the trigger springs to life. It updates the total_num
column in the resources table automatically, so you never have to worry about data inconsistency.
Here’s a glimpse at how this works in practice:
CREATE TRIGGER update_total_num
AFTER INSERT ON articles
FOR EACH ROW
EXECUTE FUNCTION update_resource_count();
This trigger is tied to the articles table. When a new article is inserted, it calls a function—update_resource_count()
—to refresh the count in the resources table. Similar triggers are set up for each resource table, so every addition is meticulously accounted for.
1400+ Free HTML Templates
271+ Free News Articles
58+ Free AI Prompts
210+ Free Code Libraries
37+ Free Code Snippets & Boilerplates for Node, Nuxt, Vue, and more!
24+ Free Open Source Icon Libraries
The Master Function: Keeping Everything in Sync
The true hero here is our update_resource_count()
function. It’s the central piece that updates the total_num
column, ensuring our master table stays accurate. Here’s a simplified version of what it might look like:
CREATE OR REPLACE FUNCTION update_resource_count()
RETURNS TRIGGER AS $$
BEGIN
UPDATE resources
SET total_num = (SELECT COUNT(*) FROM articles)
WHERE resource_name = 'articles';
RETURN NEW;
END;
$$ LANGUAGE plpgsql;
This function does the following:
- Queries the Count: It calculates the total number of entries in the articles table.
- Updates the Master Table: It sets the
total_num
in the resources table to match the newly calculated count. - Runs Automatically: Thanks to the trigger, this function is executed whenever a new article is added, keeping everything beautifully in sync.
Of course, similar functions and triggers are created for each of the other resource tables—boilerplates, templates, and so on. The setup is consistent, reliable, and ensures our data is always up to date.
Why This Setup Works Like a Charm
There’s an elegance in this system. By using Postgres triggers, we offload the responsibility of maintaining accurate counts from developers and place it squarely in the database’s capable hands. The benefits are clear:
- Data Integrity: With automatic updates, there’s no risk of
total_num
falling out of sync. - Efficiency: The system runs seamlessly in the background, with no need for manual intervention.
- Scalability: As the number of resources grows, the setup remains robust and efficient.
In essence, Postgres triggers give us peace of mind. They work tirelessly, ensuring our catalog stays accurate, even as new resources flood in.
The Takeaway: Automation Meets Simplicity
Data management doesn’t have to be a chore. With Postgres triggers, you can set up an automated system that feels almost magical, keeping everything in sync without breaking a sweat. So, the next time you think about adding a new resource type, you’ll know your database is ready to handle it with grace and precision.
In the world of data, automation is king—and Postgres triggers are the knights that make it all happen.
For more tips on web development, check out DailySandbox and sign up for our free newsletter to stay ahead of the curve!
Comments ()