How to Use Object.groupBy() in JavaScript: A Quick Guide
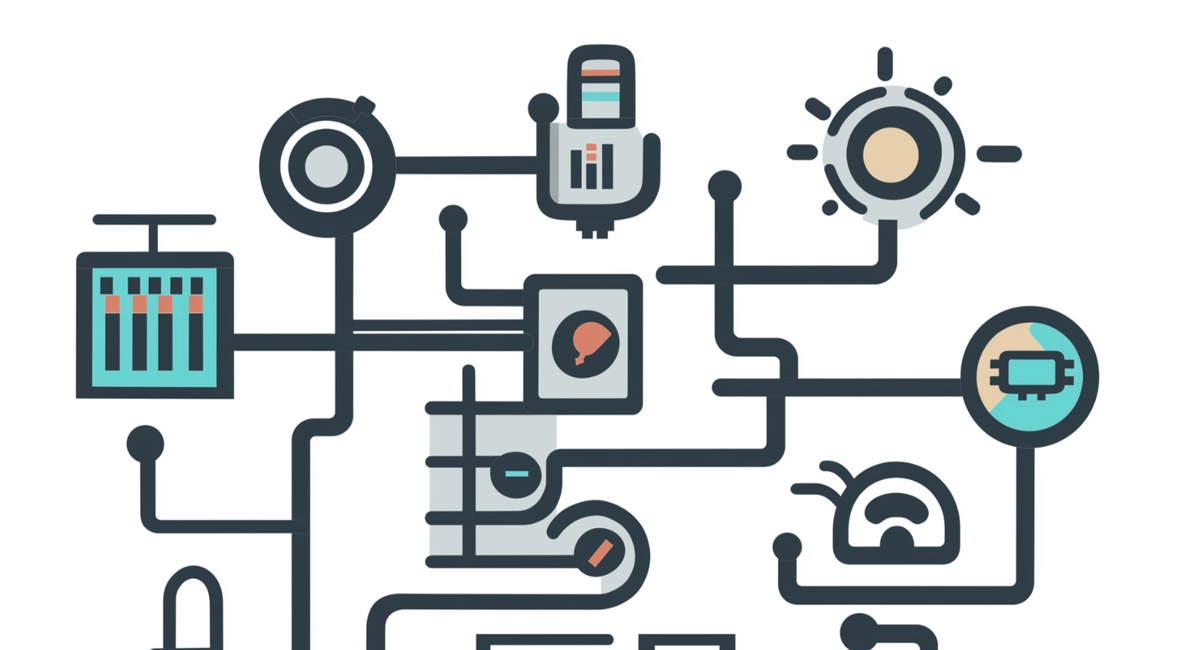
Unlocking Data Organization with JavaScript's Object.groupBy()
JavaScript developers, rejoice! ECMAScript 2024 brings us Object.groupBy()
, a feature that makes grouping data easier, faster, and more intuitive than ever. This powerful new tool lets you organize arrays with minimal code, transforming the way you handle data.
What’s the Big Deal About Object.groupBy()
?
Imagine having an array of data you want to categorize—maybe numbers by parity, items by category, or events by year. Previously, grouping this data meant writing custom functions and loops. Now, with Object.groupBy()
, you can achieve the same results with a single line of code.
The Magic Syntax
At its core, Object.groupBy()
takes an array and a grouping function that defines how to categorize each element:
Object.groupBy(array, groupingFunction);
Here, groupingFunction
returns the key each item belongs to, creating an object where each key holds an array of matching items. Simple, clean, and effective.
Example 1: Grouping Numbers by Even and Odd
Let’s start with something classic: separating numbers into even and odd groups.
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const groupedNumbers = Object.groupBy(numbers, num => num % 2 === 0 ? 'even' : 'odd');
console.log(groupedNumbers);
Output:
{
even: [2, 4, 6, 8, 10],
odd: [1, 3, 5, 7, 9]
}
With just a single line, we’ve neatly organized numbers into even and odd categories. No fuss, no custom functions—just a clean, declarative grouping.
1391+ Free HTML Templates
271+ Free News Articles
49+ Free AI Prompts
210+ Free Code Libraries
37+ Free Code Snippets & Boilerplates for Node, Nuxt, Vue, and more!
24+ Free Open Source Icon Libraries
Example 2: Grouping Objects by Category
Now, imagine you have a list of products you want to categorize. Object.groupBy()
lets you do this effortlessly:
const products = [
{ name: 'Laptop', category: 'Electronics' },
{ name: 'Shirt', category: 'Clothing' },
{ name: 'Phone', category: 'Electronics' },
{ name: 'Jeans', category: 'Clothing' },
];
const groupedProducts = Object.groupBy(products, product => product.category);
console.log(groupedProducts);
Output:
{
Electronics: [
{ name: 'Laptop', category: 'Electronics' },
{ name: 'Phone', category: 'Electronics' }
],
Clothing: [
{ name: 'Shirt', category: 'Clothing' },
{ name: 'Jeans', category: 'Clothing' }
]
}
This example highlights how Object.groupBy()
streamlines complex data organization, making it easy to group products by category with minimal code.
Example 3: Organizing Events by Year
Let’s level up with a date-based example, grouping events by the year they occur. With Object.groupBy()
, organizing items based on derived data—like years—is a breeze:
const events = [
{ title: 'Conference', date: '2024-06-12' },
{ title: 'Meeting', date: '2023-08-21' },
{ title: 'Workshop', date: '2024-09-05' },
{ title: 'Webinar', date: '2023-11-18' }
];
const groupedEvents = Object.groupBy(events, event => new Date(event.date).getFullYear());
console.log(groupedEvents);
Output:
{
2024: [
{ title: 'Conference', date: '2024-06-12' },
{ title: 'Workshop', date: '2024-09-05' }
],
2023: [
{ title: 'Meeting', date: '2023-08-21' },
{ title: 'Webinar', date: '2023-11-18' }
]
}
By grouping events by year, we’re able to manage and visualize data across time—an invaluable tool for organizing timelines, appointments, or project milestones.
Why Object.groupBy()
is a Game-Changer
- Elegance and Readability: Say goodbye to sprawling code and custom grouping functions.
- Performance:
Object.groupBy()
leverages JavaScript’s native capabilities, making it efficient. - Versatility: From basic lists to complex datasets,
Object.groupBy()
adapts effortlessly.
Embrace the Future of JavaScript
With Object.groupBy()
, JavaScript developers have a new ally for cleaner, smarter code. ECMAScript 2024 is making grouping as intuitive as it should be, letting us spend more time on logic and less on boilerplate. Dive into Object.groupBy()
today and experience the difference—your code (and your future self) will thank you!
For more tips on web development, check out DailySandbox and sign up for our free newsletter to stay ahead of the curve!
Comments ()