How to encourage signups with a "Join the Club" modal and fading content
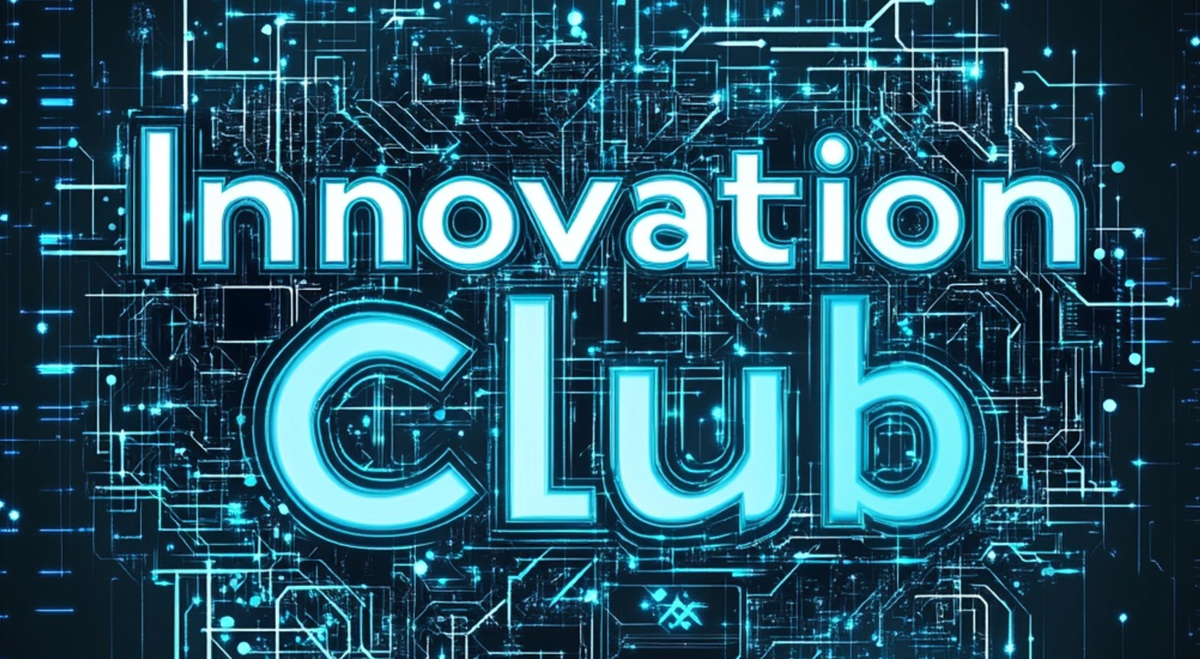
Imagine browsing a website, catching a glimpse of intriguing content just out of reach, with a simple modal enticing you to "join the club" for unlimited access. This subtle yet effective design piques curiosity while encouraging action. In this tutorial, we’ll build such an experience using PrimeVue’s Dialog component in Nuxt 3, complete with a graceful content fade effect that draws users in.
Note: This could just as easily be designed in vanilla JS, or without using PrimeVue.
Let’s dive into crafting this captivating modal experience while focusing on its psychological effectiveness—letting users preview a snippet of content to make joining the club irresistible.
Part 1: The Purpose and Setup
The goal is simple: when a user isn’t logged in, display a "Join the Club" modal while fading the background content to hint at what lies beneath. This technique leverages curiosity, a powerful motivator, to encourage sign-ups.
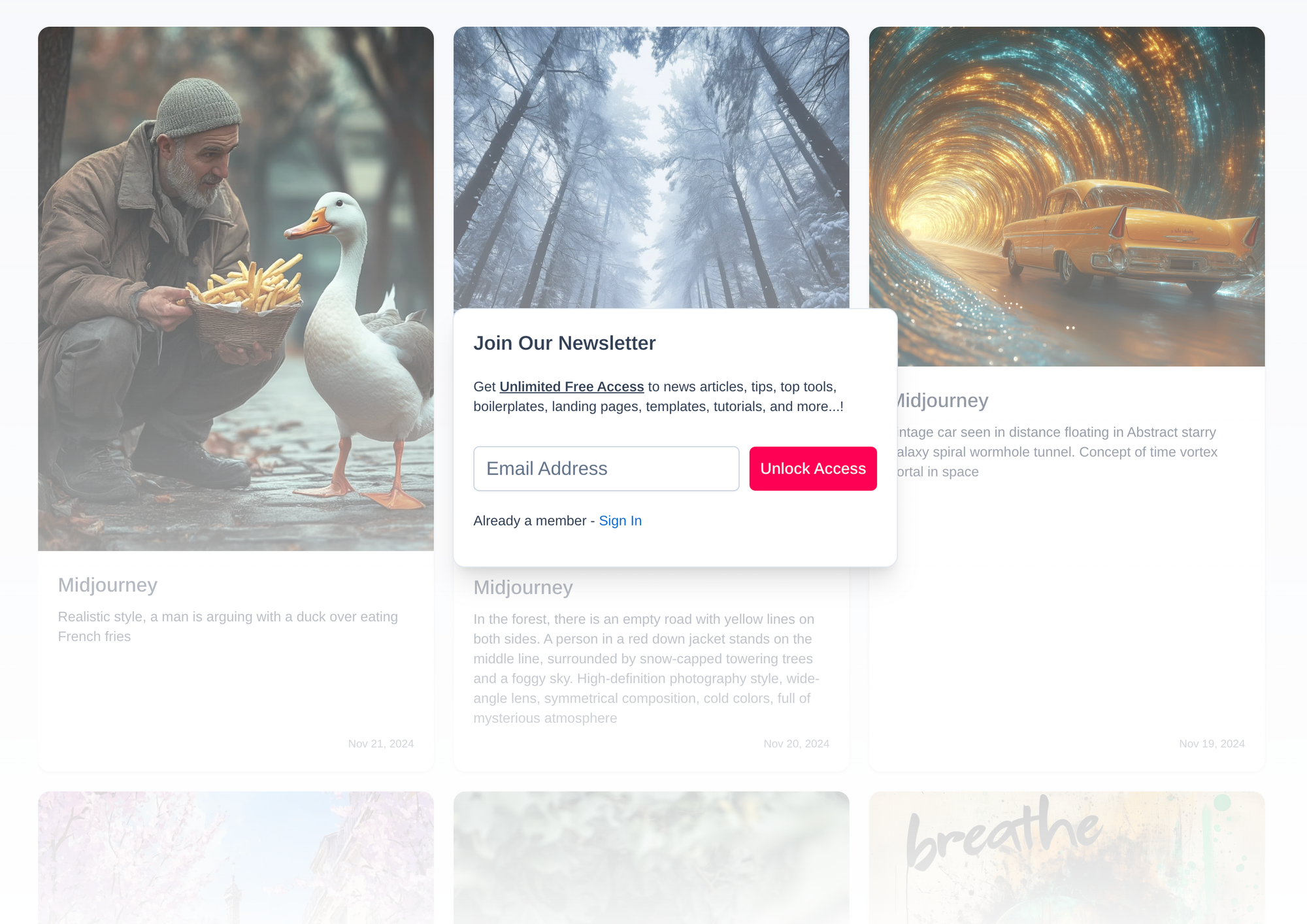
Initializing the Component
Create a join-the-club.vue
file and set up the basic script and template:
<script setup>
const showLoginDialog = ref(true); // Controls the modal visibility
const email = ref(''); // Holds the user's email input
// Dynamic body class to manage overflow
const body_class = computed(() => ({
overflow: showLoginDialog.value,
}));
// Join the club function (placeholder for now)
const joinClub = async () => {
console.log('User email:', email.value);
};
// Placeholder function for sign-in click
const onSigninClicked = (event) => {
console.log('Sign-in clicked');
};
</script>
Here, we define:
showLoginDialog
: A reactive variable that determines whether the modal is visible.email
: A reactive variable to capture the user’s email input.joinClub
andonSigninClicked
: Placeholder functions for handling actions.
Part 2: Crafting the Modal
Using PrimeVue’s Dialog component, we’ll create a modal that’s elegant, non-intrusive, and purpose-driven. The modal provides a clear call to action and simplifies the decision-making process.
Adding the Template
<template>
<Body :class="body_class" />
<!-- Background overlay with fade effect -->
<div v-if="showLoginDialog" class="content-auth-overlay" />
<!-- PrimeVue Dialog -->
<Dialog
v-model:visible="showLoginDialog"
header="Join The Club"
:draggable="false"
:closable="false"
:dismissable-mask="false"
:close-on-escape="false"
:style="{ width:'450px' }"
>
<p>
Get <u><strong>Unlimited Free Access</strong></u> to everything we have to offer, and more...!
</p>
<!-- Email input and button -->
<div class="join-club">
<InputText
ref="emailRef"
v-model="email"
class="email-input"
placeholder="Email Address"
@keydown.enter="joinClub"
/>
<Button
label="Join Now"
class="email-control"
@click="joinClub"
/>
</div>
<p>Already a member? <a href="#" @click="onSigninClicked">Sign In</a></p>
</Dialog>
</template>
- Content Preview: The gradient overlay provides a teaser of what’s underneath, enticing the user to explore.
- PrimeVue Dialog: This non-dismissable modal focuses the user’s attention while still being friendly.
1400+ Free HTML Templates
351+ Free News Articles
67+ Free AI Prompts
315+ Free Code Libraries
52+ Free Code Snippets & Boilerplates for Node, Nuxt, Vue, and more!
25+ Free Open Source Icon Libraries
Part 3: Styling for Engagement
Great functionality deserves great styling. Let’s add CSS to enhance the user experience.
Styling the Overlay and Modal
<style lang="less" scoped>
.content-auth-overlay {
position: fixed;
top: 55px;
bottom: 0;
left: 0;
right: 0;
width: 100%;
height: 100%;
background: linear-gradient(to bottom, rgba(255, 255, 255, 10%), rgba(255, 255, 255, 100%));
z-index: 1000;
pointer-events: all;
opacity: 1;
}
.join-club {
display: flex;
align-items: center;
margin-top: 30px;
margin-bottom: 20px;
width: 100%;
@media @mobile {
flex-flow: column;
align-items: normal;
gap: 15px;
}
}
.email-input {
font-size: 1.2rem;
}
.email-control {
font-size: 1rem;
white-space: nowrap;
overflow: unset;
padding: 11px;
margin-left: 10px;
}
</style>
- Overlay Effect: The
linear-gradient
creates a fade-out effect, leaving just enough visibility to intrigue the user. - Responsive Design: Mobile-first adjustments ensure the layout works across devices.
- Styling Inputs: Clean and modern input and button designs enhance usability.
Part 4: Adding Functionality
The joinClub
function is the heart of this modal. It will handle user email submissions and trigger backend logic for sign-ups.
Adding the Join Functionality
const joinClub = async () => {
if (!email.value) {
console.error('Email is required');
return;
}
try {
// Simulate a call to the backend API
console.log('Joining the club with email:', email.value);
showLoginDialog.value = false; // Close the modal on success
} catch (error) {
console.error('Error joining the club:', error);
}
};
- Validation: Ensure the email is provided before proceeding.
- Simulated Backend Call: Replace the
console.log
with an actual API call to handle sign-ups. - Closing the Modal: Upon success, hide the modal to let the user explore the site.
Part 5: Tying It All Together
Now, integrate the join-the-club.vue
component into your main app. For instance, you can import and use it conditionally based on the user’s authentication state:
<template>
<div>
<join-the-club v-if="!userLoggedIn" />
<main-content v-else />
</div>
</template>
<script setup>
import JoinTheClub from '~/components/join-the-club.vue';
const userLoggedIn = false; // Replace with actual authentication state
</script>
The Psychology of the Fade Effect
This design leverages a powerful principle of curiosity. By allowing users to glimpse part of the content beneath the modal, you tap into their desire to discover what they’re missing. Coupled with the clear value proposition in the modal text, this approach encourages users to make quick decisions, increasing conversions.
Conclusion: More Than a Modal
With this setup, you’ve created more than just a "Join the Club" modal. You’ve crafted a persuasive and thoughtful experience that combines visual appeal with user psychology to drive engagement. The PrimeVue Dialog and the gradient overlay work in harmony to captivate your audience while providing an intuitive and responsive interface.
Stay tuned for more in this series as we continue to build engaging features that delight users and elevate your web applications!
For more tips on web development, check out DailySandbox and sign up for our free newsletter to stay ahead of the curve!
Comments ()