Part 1 - Building a RESTful API with Node.js and Express
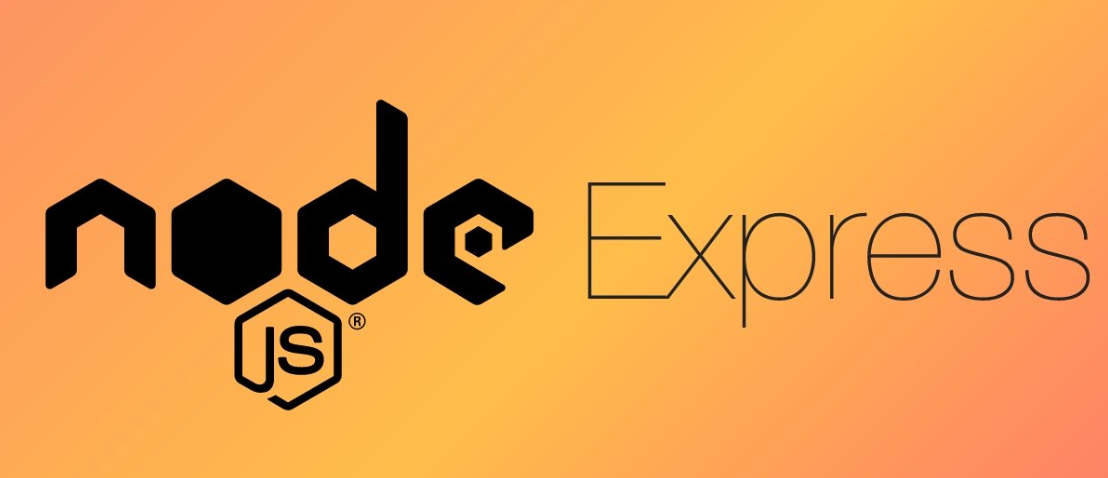
Imagine a bustling marketplace, where vendors are ready to serve your every need with precision and speed. That’s what a RESTful API is like—a well-organized system that lets your applications communicate seamlessly, like shoppers finding exactly what they need, when they need it. And at the heart of this digital marketplace? Node.js and Express, the dynamic duo that makes setting up an API feel like crafting a piece of art.
If you’ve ever felt intimidated by the idea of building your own API, fear not. In this tale, we’ll walk through setting up a RESTful API that’s efficient, flexible, and easy to maintain. Think of it as building your own digital bazaar, where each endpoint is a vendor, ready to deliver data or handle requests with grace.
The Setup: Starting with Node.js and Express
Before we dive into writing code, we need to set up our environment. Node.js, a powerhouse built on Chrome’s V8 engine, is our foundation. Express, a minimalist web framework for Node.js, is the scaffolding that makes constructing our API a breeze.
Step 1: Initialize Your Project
Fire up your terminal and create a new project directory:
mkdir restful-api
cd restful-api
Next, initialize your project with npm
to create a package.json
file:
npm init -y
This file is your project’s manifesto, containing metadata about your API, dependencies, and scripts. Think of it as a table of contents for your digital bazaar.
Step 2: Installing Dependencies
Now, let’s bring in Express to help us manage our endpoints. We’ll also install nodemon
, a handy tool that automatically restarts the server when you make changes:
npm install express
npm install --save-dev nodemon
With these tools in place, you’re ready to start building.
1400+ Free HTML Templates
325+ Free News Articles
62+ Free AI Prompts
292+ Free Code Libraries
51+ Free Code Snippets & Boilerplates for Node, Nuxt, Vue, and more!
25+ Free Open Source Icon Libraries
The Blueprint: Setting Up Your API
Our goal is to create a simple RESTful API that handles CRUD operations (Create, Read, Update, Delete). Think of it as a marketplace where you can add new vendors (data), retrieve information about them, update their details, or remove them entirely.
Step 3: Creating Your Server
Create a file named app.js
in your project directory. Here’s where we’ll write the code for our API:
const express = require('express');
const app = express();
const port = 3000;
// Middleware to parse JSON
app.use(express.json());
// A simple "Hello, world!" route
app.get('/', (req, res) => {
res.send('Welcome to our RESTful API!');
});
// Start the server
app.listen(port, () => {
console.log(`API is running on http://localhost:${port}`);
});
And just like that, you have a basic Express server up and running. Launch it with:
nodemon app.js
nodemon
watches for changes in your code and automatically restarts the server, saving you from manual restarts. It’s like having a helpful assistant who keeps everything running smoothly.
Building the Marketplace: CRUD Endpoints
Now, let’s flesh out our API to handle CRUD operations. Imagine we’re managing a collection of vendors, each represented by a simple JavaScript object.
Step 4: Setting Up Your Data
For simplicity, we’ll use an in-memory array to store our vendor data. In a real-world scenario, you’d use a database, but for now, this will do:
let vendors = [
{ id: 1, name: 'Vendor One', specialty: 'Fruit' },
{ id: 2, name: 'Vendor Two', specialty: 'Vegetables' }
];
Step 5: Creating CRUD Routes
Now comes the fun part: setting up our endpoints. Each route will correspond to a different CRUD operation.
- Create: Add a new vendor to the marketplace.
- Read: Retrieve information about one or all vendors.
- Update: Modify an existing vendor’s details.
- Delete: Remove a vendor from the marketplace.
Here’s how it all comes together:
// Create: Add a new vendor
app.post('/vendors', (req, res) => {
const newVendor = {
id: vendors.length + 1,
name: req.body.name,
specialty: req.body.specialty
};
vendors.push(newVendor);
res.status(201).json(newVendor);
});
// Read: Get all vendors
app.get('/vendors', (req, res) => {
res.json(vendors);
});
// Read: Get a single vendor by ID
app.get('/vendors/:id', (req, res) => {
const vendor = vendors.find(v => v.id === parseInt(req.params.id));
if (!vendor) return res.status(404).send('Vendor not found');
res.json(vendor);
});
// Update: Modify an existing vendor
app.put('/vendors/:id', (req, res) => {
const vendor = vendors.find(v => v.id === parseInt(req.params.id));
if (!vendor) return res.status(404).send('Vendor not found');
vendor.name = req.body.name || vendor.name;
vendor.specialty = req.body.specialty || vendor.specialty;
res.json(vendor);
});
// Delete: Remove a vendor
app.delete('/vendors/:id', (req, res) => {
const vendorIndex = vendors.findIndex(v => v.id === parseInt(req.params.id));
if (vendorIndex === -1) return res.status(404).send('Vendor not found');
vendors.splice(vendorIndex, 1);
res.status(204).send();
});
Each of these routes serves a specific purpose, like different stalls in our marketplace. We’re using HTTP methods (POST
, GET
, PUT
, DELETE
) to ensure our API adheres to RESTful conventions.
The Benefits of Using Node.js and Express
Why choose Node.js and Express for your API? It’s simple: they’re fast, efficient, and incredibly developer-friendly. Here’s why:
- Speed and Performance: Node.js uses a non-blocking, event-driven architecture, making it perfect for real-time applications and APIs that need to handle a high volume of requests.
- Ease of Use: Express makes building APIs a breeze, with intuitive methods and a minimalist approach that doesn’t get in your way.
- Scalability: As your marketplace grows, so can your API. Node.js and Express are designed to scale, handling increased traffic without breaking a sweat.
The Finale: Running Your API
With everything in place, your RESTful API is ready to serve data to the world. Fire it up, make some requests using your favorite tool (like Postman or cURL), and watch as your marketplace of data comes to life.
And there you have it: a RESTful API built with Node.js and Express, efficient and elegant. Like a master craftsman, you’ve constructed a digital marketplace that’s flexible, robust, and ready for action. So go ahead—celebrate your creation, and remember: in the world of APIs, simplicity and power can go hand in hand.
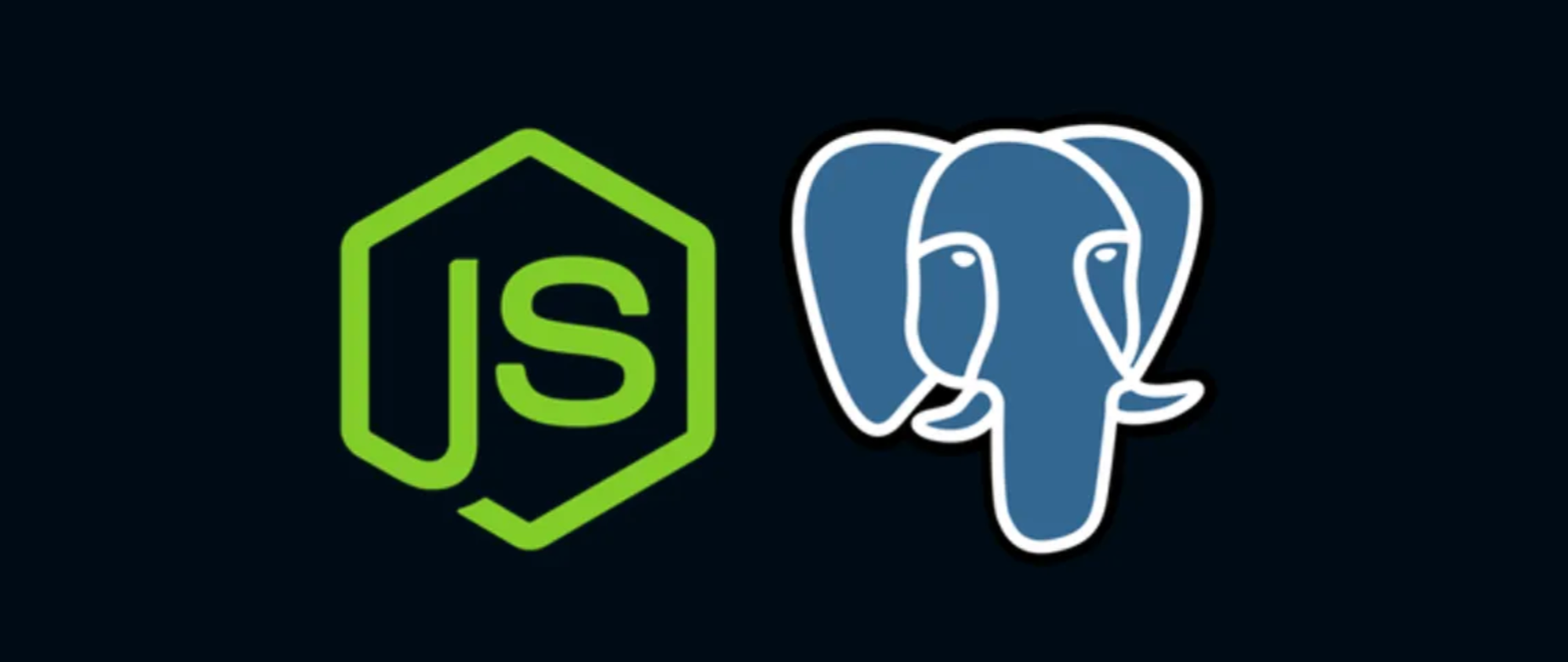
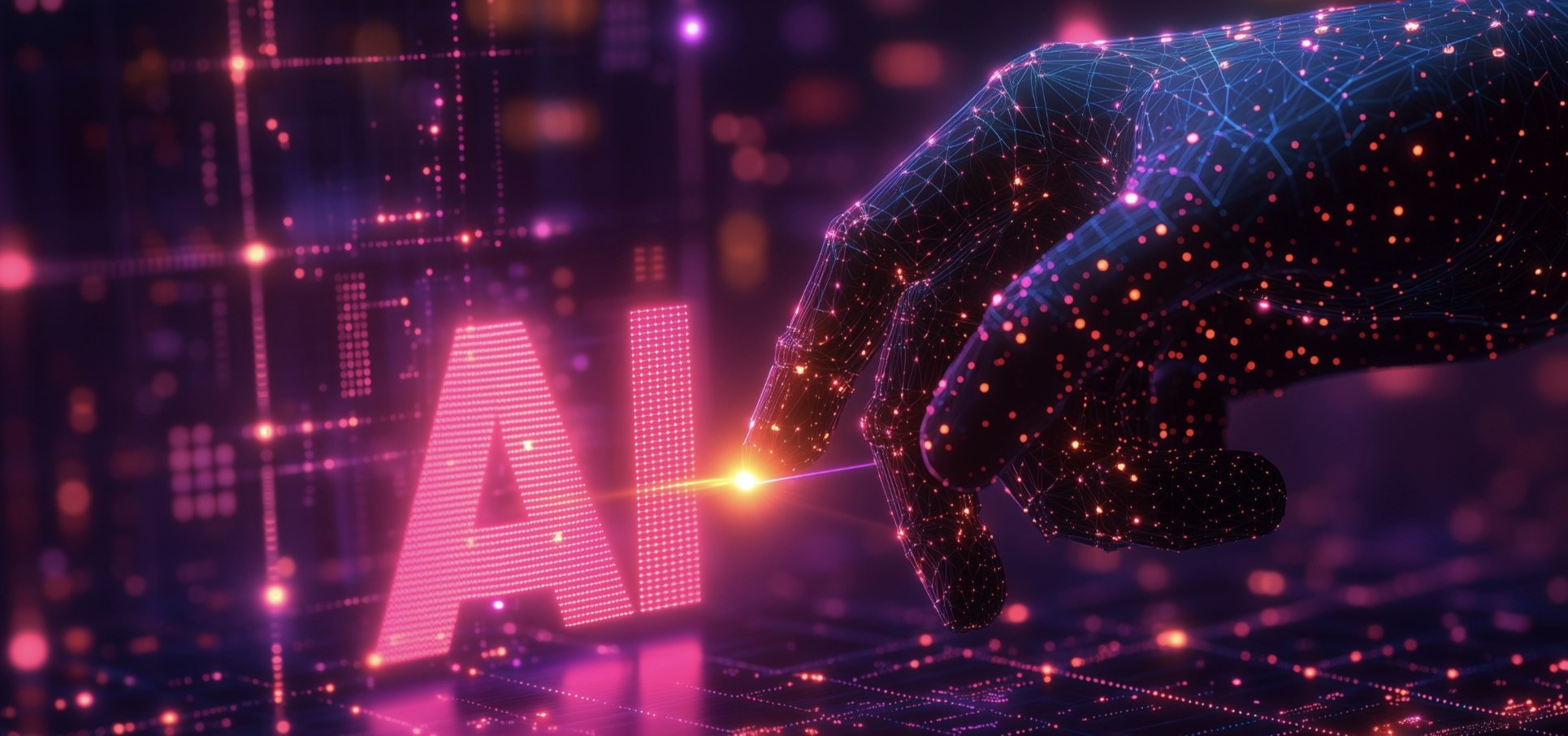
For more tips on web development, check out DailySandbox and sign up for our free newsletter to stay ahead of the curve!
Comments ()